Have you ever wanted to ensure your customers know the shipping cost before adding a product to their cart? It could help reduce cart abandonment.
To solve this problem, you can display shipping costs directly on the product page. In this tutorial, I’ll show you how to do it easily.
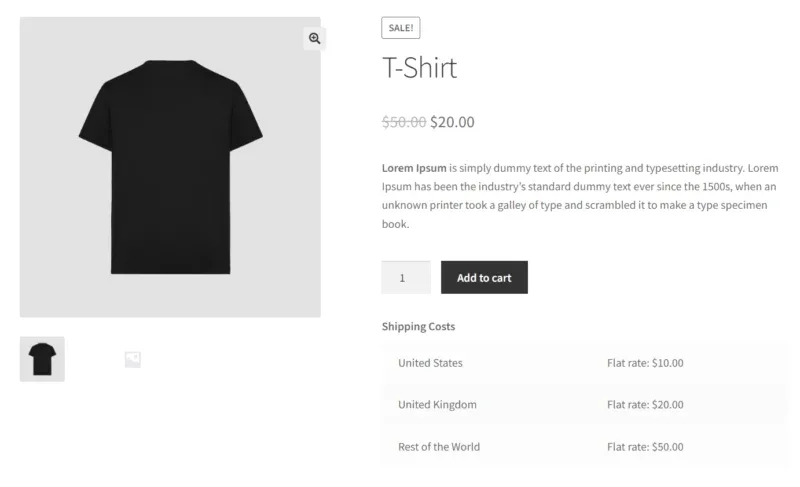
Table of Contents
Enable Shipping Zones and Methods
To display shipping costs on product pages, you must set up your shipping zones and methods properly. If you haven’t set up shipping yet:
- Go to WooCommerce > Settings > Shipping.
- Click “Add Shipping Zone” if you don’t have one already.
- Add shipping methods like Flat Rate, Free Shipping, or Local Pickup under each zone.
Code Snippet to Display Shipping Costs on Product Pages
The code shows a list of all available shipping zones. It includes the zone names, shipping types, and costs for each zone.
Be sure to use clear names when creating your shipping zones. These names will appear in the list.
If a shipping zone is created but has no shipping methods set up, it will not be included in the list shown to customers.
If you want to change the list label, modify this line:. __( 'Shipping Costs', 'woocommerce' ) . '</strong><br>';
You can also use the .shipping-cost-info
class to customize the styling of the section.
/**
* Snippet: Display Shipping Costs on the Product Pages
* Author: Toufique Alahi
* URL: https://wpblogr.com
* Tested with WooCommerce 9.3.3
*/
add_action( 'woocommerce_after_add_to_cart_button', 'wpblogr_display_shipping_costs_below_cart_button', 20 );
function wpblogr_display_shipping_costs_below_cart_button() {
global $product;
// Ensure the product requires shipping
if ( ! $product->needs_shipping() ) return;
// Add the top margin for the container and the margin for the table
echo '<div style="margin-top: 2em;" class="shipping-cost-info"><strong>'
. __( 'Shipping Costs', 'woocommerce' ) . '</strong><br>';
echo '<table style="margin-top: 0.5em; margin-bottom: 0;">';
// Fetch all defined shipping zones
$shipping_zones = WC_Shipping_Zones::get_zones();
foreach ( $shipping_zones as $zone_id => $zone ) {
// Check if the zone has any enabled shipping methods
$active_methods = array_filter( $zone['shipping_methods'], function( $method ) {
return $method->enabled === 'yes';
});
// Skip the zone if no active methods
if ( empty( $active_methods ) ) continue;
echo '<tr><td>' . esc_html( $zone['zone_name'] ) . '</td><td>';
// Display each active method with valid cost
foreach ( $active_methods as $method ) {
$method_title = $method->get_method_title();
$method_cost = isset( $method->cost ) && $method->cost !== '' ? wc_price( $method->cost ) : __( 'N/A', 'woocommerce' );
echo esc_html( $method_title ) . ': ' . $method_cost . '<br>';
}
echo '</td></tr>';
}
// Code to Include the "Rest of the World" zone
$default_zone = new WC_Shipping_Zone( 0 ); // 0 is the ID for Rest of the World zone
$default_methods = array_filter( $default_zone->get_shipping_methods(), function( $method ) {
return $method->enabled === 'yes';
});
if ( ! empty( $default_methods ) ) {
echo '<tr><td>' . __( 'Rest of the World', 'woocommerce' ) . '</td><td>';
foreach ( $default_methods as $method ) {
$method_title = $method->get_method_title();
$method_cost = isset( $method->cost ) && $method->cost !== '' ? wc_price( $method->cost ) : __( 'N/A', 'woocommerce' );
echo esc_html( $method_title ) . ': ' . $method_cost . '<br>';
}
echo '</td></tr>';
}
// End of Code for "Rest of the World" zone
echo '</table></div>';
}
How to Add the Code Snippet
You should add the code snippet to the functions.php
file in your child theme. If you don’t have a child theme, read this guide on How to Create a Child Theme.
Once you have the child theme, follow these steps:
- Go to your WordPress dashboard.
- Hover over Appearance and click on Theme File Editor.
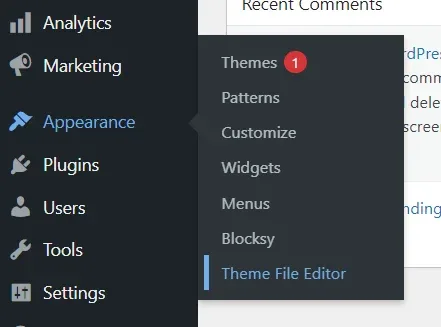
- On the right side, find
functions.php
andstyle.css
- Click on file names to add the code.
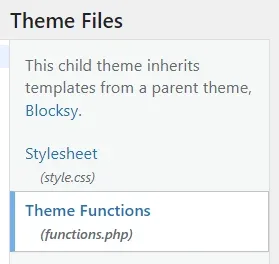
Paste the code and click on Update File.
If you have any questions, feel free to leave a comment below. I will try my best to answer.